Extension 5.1: Chase, The Mouse (6 points)¶
A Story¶
Chase, the Mouse, was standing still on the Standard Draw template one day, thinking about the meaning of life. All of a sudden, out of nowhere, a ball began to follow Chase. No matter where Chase went the ball would follow and pester Chase until he moved again. You will be writing the code for the ball.
Procedure¶
Open
ChaseTheMouse.java
in themousechaser
package in the src folder. You will have to complete several methods for this extension.Note
X
andY
.
private static final int X = 0;
private static final int Y = 1;
Use these to index the X and Y coordinates of the points represented as double arrays of length 2 in this extension.
Complete
getMousePosition()
.Return a created array of length 2 with the array item at the X index assigned to StdDraw.mouseX() and the array item at the Y index assigned to StdDraw.mouseY().
Run
MousePositionDebugApp
as a Java Application.
Complete
drawBall(location, radius)
Run
DrawBallDebugApp
as a Java Application.
Complete
square(v)
.Question: What is a simple way to implement squaring a number without calling
Math.pow(x,y)
?Run
ChaseTheMouseTestSuite
as a JUnit Test.
Complete
calculateMagnitude(xy)
.Vector Magnitude on Wolfram MathWorld
Note: vector
xy
only has 2 dimensions.Run
ChaseTheMouseTestSuite
.Make any necessary edits to calculateMagnitude until the CalculateMagnitude test cases have all passed.
Complete
add(a, b)
.Vector Addition on Wolfram MathWorld
Note: vectors
a
andb
only have 2 dimensions.Run
ChaseTheMouseTestSuite
.Make any necessary edits to add until the Add test cases have all passed.
Complete
subtract(a, b)
.Vector Subtraction on Wolfram MathWorld
Note: vectors
a
andb
only have 2 dimensions.Run
ChaseTheMouseTestSuite
.Make any necessary edits to subtract until the Subtract test cases have all passed.
Complete
scalarMultiply(xy, scalar)
.Scalar Multiplication on Wolfram MathWorld
Scalar Multiplication on Wikipedia
Note: vector
xy
only has 2 dimensions.Run
ChaseTheMouseTestSuite
.Make any necessary edits to scalarMultiply until the ScalarMultiply test cases have all passed.
Watch this video on Real Time Animation
Complete
calculateNextPosition(currentBallPosition, mousePosition, speed, deltaTime)
.Tip: Use the utility methods you have writen above to implement this method.
Rather than the speed of the animation being dependent on how fast our loop executes, we are going to keep track of our time to ensure that our ball always moves at a constant speed.
Below are two different images which attempt to convey what is required:
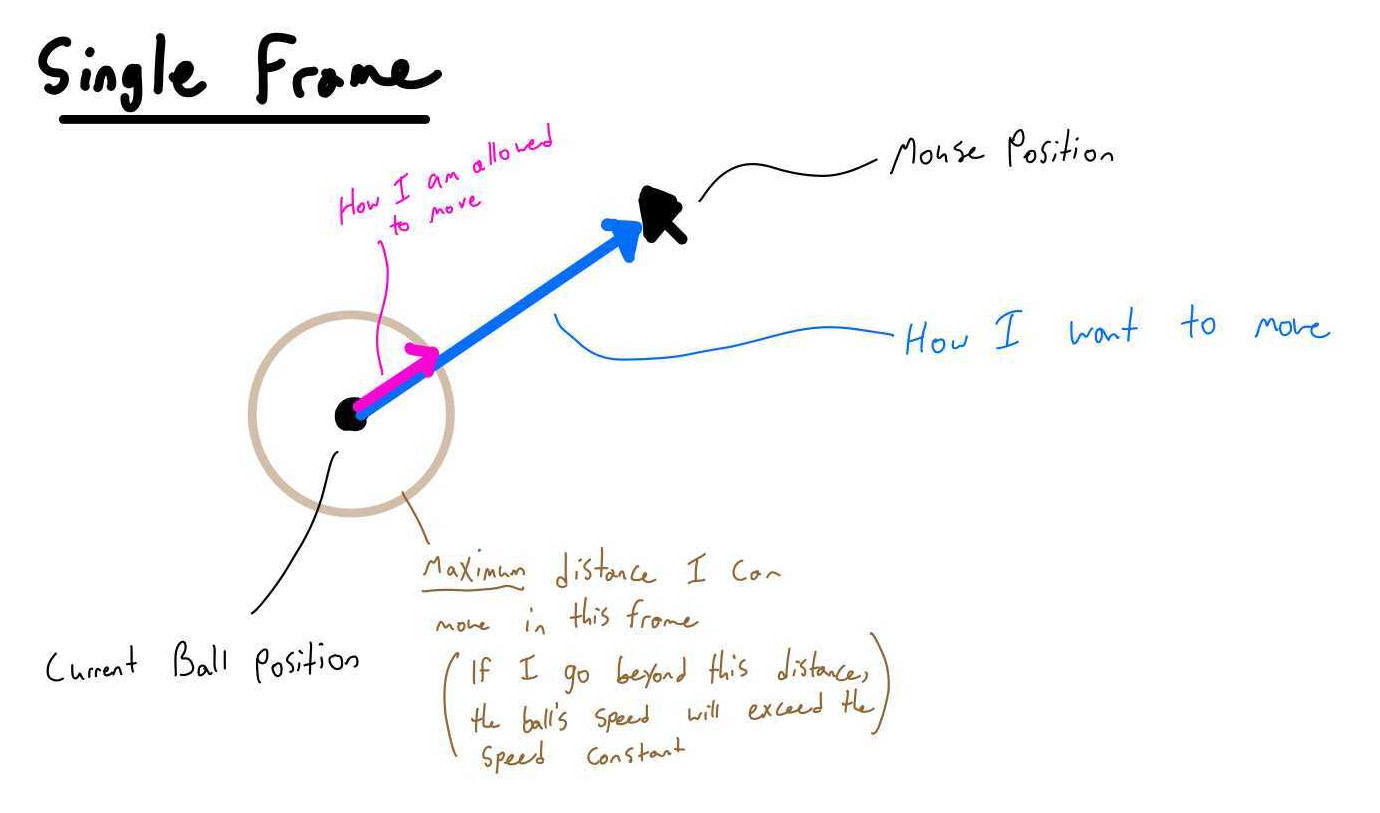
Run
ChaseTheMouseTestSuite
.assertArrayEquals(contentsMessage, expected, actual, 0.0001);
Make any necessary edits to calculateNextPosition until the CalculateNextPosition test cases have all passed.
Run
CalculateNextPositionDebugApp
as a Java Application.
Complete
chaseTheMouse()
Tip: Use
Timing.getCurrentTimeInSeconds()
to… well… get the current time in seconds. Within your loop, keep track of the previous time to calculate the change in time since the previous iteration of the loop.Run
ChaseTheMouse
as a Java Application