Multi-Selection: else-if Statements¶
Using if/else statements, you can even pick between 3 or more possibilites. Just add else if for each possibility after the first if, and else before the last possibility.
1// 3 way choice with else if
2if (boolean expression) {
3 statement1;
4} else if (boolean expression) {
5 statement2;
6} else {
7 statement3;
8}
Run the
E01TestElseIf
program and try changing the value of x to get each of the three possible lines in the conditional to print.
Here is a flowchart for a conditional with 3 options like in the code above.
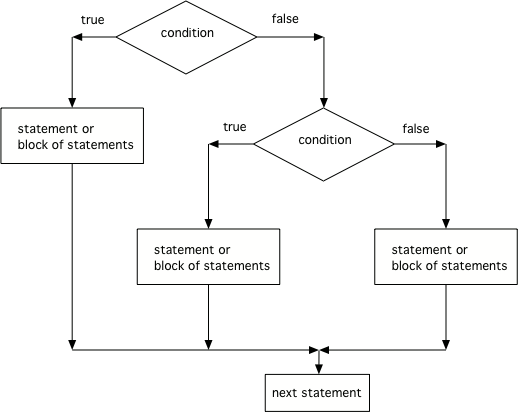
Figure 1: The order that statements execute in a conditional with 3 options: if, else if, and else¶
Note
Another way to handle 3 or more conditional cases is to use the switch
and break
keywords. For a tutorial on using switch see https://docs.oracle.com/javase/tutorial/java/nutsandbolts/switch.html.
- x is negative
- When x is equal to -5 the condition of x < 0 is true.
- x is zero
- This will only print if x has been set to 0. Has it?
- x is positive
- This will only print if x is greater than zero. Is it?
3-4-1: What does the following code print when x has been set to -5?
1if (x < 0) {
2 System.out.println("x is negative");
3} else if (x == 0) {
4 System.out.println("x is zero");
5} else {
6 System.out.println("x is positive");
7}
- x is negative
- This will only print if x has been set to a number less than zero. Has it?
- x is zero
- This will only print if x has been set to 0. Has it?
- x is positive
- The first condition is false and x is not equal to zero so the else will execute.
3-4-2: What does the following code print when x has been set to 2000?
1if (x < 0) {
2 System.out.println("x is negative");
3} else if (x == 0) {
4 System.out.println("x is zero");
5} else {
6 System.out.println("x is positive");
7}
- first quartile
- This will only print if x is less than 0.25.
- second quartile
- This will only print if x is greater than or equal to 0.25 and less than 0.5.
- third quartile
- The first only print if x is greater than or equal to 0.5 and less than 0.75.
- fourth quartile
- This will print whenever x is greater than or equal to 0.75.
3-4-3: What does the following code print when x has been set to .8?
1if (x < .25) {
2 System.out.println("first quartile");
3} else if (x < .5) {
4 System.out.println("second quartile");
5} else if (x < .75) {
6 System.out.println("third quartile");
7} else {
8 System.out.println("fourth quartile");
9}
The else-if connection is necessary if you want to hook up conditionals together. In the
E02IfDebug
program, there are 4 separate if statements instead of the if-else-if pattern. Will this code print out the correct grade? First, trace through the code to see why it prints out the incorrect grade, using the debugger. Then, fix the code by adding in 3 else’s to connect the if statements and see if it works.Finish the
E03BatteryTest
program so that it prints “Plug in your phone!” if the battery is below 50, “Unplug your phone!” if it is above 100, and “All okay!” otherwise. Change the battery value to test all 3 conditions.
Summary¶
A multi-way selection is written when there are a series of conditions with different statements for each condition.
Multi-way selection is performed using if-else-if statements such that exactly one section of code is executed based on the first condition that evaluates to true.
1// 3 way choice with else if
2if (boolean expression) {
3 statement1;
4} else if (boolean expression) {
5 statement2;
6} else {
7 statement3;
8}